In the realm of web development, CSS stands as a fundamental pillar, offering developers the power to dictate the presentation and structure of web content, including the styling of buttons. By selecting HTML elements and applying rules, CSS defines the visual appeal of various components on a webpage. One crucial aspect is centering buttons, a skill that significantly influences the aesthetics and user experience of a website. Precise button alignment can elevate user engagement and contribute to an aesthetically pleasing design.
What are CSS Buttons?
A CSS button serves as an interactive element on a webpage, typically utilized to initiate actions like form submission or page navigation. These buttons are crafted using the HTML button element and are customized in terms of appearance and behavior through CSS properties.
Creating a CSS Button
To create a CSS button, you first define a button element in your HTML and then assign a class to it. This class is then employed in your CSS stylesheet to style the button. For instance:
<button type="button" class="button">Click me!</button>
In the given example, the “button” class is used to apply styles to the button from a separate CSS file. You can choose any other name for the class, such as “btn.”
Diverse Properties of a CSS Button
CSS offers a broad array of properties that can be utilized to manage the appearance and functionality of buttons. Here are some commonly used properties:
- background-color: Alters the background color of a button. For instance, `background-color: #04AA6D` will render the button with a green background;
- padding: Controls the spacing between the button’s content and its border. For example, padding: 15px 32px` will provide the button with 15px padding on the top and bottom, and 32px on the left and right;
- border-radius: Adds rounded corners to a button. For example, `border-radius: 10px` will give the button rounded corners with a radius of 10px;
- border: Manages the border of a button. For example, `border: 2px solid #04AA6D` will give the button a 2px solid green border;
- box-shadow: Introduces a shadow effect to a button. For example, `box-shadow: 0 8px 16px 0 rgba(0,0,0,0.2), 0 6px 20px 0 rgba(0,0,0,0.19)` will create a shadow effect for the button.
Additional Properties
Additional Properties
- opacity: This property is used to control the transparency of a button. For example, opacity: 0.5; will make the button semi-transparent;
- cursor: This property is used to change the cursor style when the mouse hovers over the button. For example, cursor: pointer; will change the cursor to a hand icon when it hovers over the button;
- width and height: These properties are used to control the size of the button. For example, width: 250px; and height: 50px; will set the width and height of the button;
- float: This property is used to control the positioning of the button. For example, float: left; will make the button float to the left of its container;
- border for button groups: This property is used to create a bordered button group. For example, border: 1px solid green; will create a bordered button group with a green border.
Below is an illustration of how the button will appear when these properties are applied:
button {
background-color: #04AA6D;
border: 2px solid #aa0462;
border-radius: 10px;
padding: 15px 32px;
display: block;
cursor: pointer;
box-shadow: 0 8px 16px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19);
width: 250px;
height: 50px;
float: left;
opacity: 0.5;
}
Methodologies for Button Centering in a Div
The task of centering a button inside a div can be approached in numerous ways. The method you prefer may hinge on the specific requirements of your design and the layout you envision for your webpage.
Method 1. Utilizing Flexbox for Button Centering
Flexbox, or the Flexible Box Layout, is a contemporary CSS design modality that allows you to create robust, fluid layouts without having to resort to float or position properties. It simplifies the positioning of elements within a container and is particularly useful for scaling interface components in responsive designs.
If you intend to center a button within a div using Flexbox, you’d need to assign the display property of the div to flex and then deploy align-items and justify-contentproperties to center the button, both vertically and horizontally.
Here’s how you can do it:
<!DOCTYPE html>
<html>
<head>
<title>Centering Button Demo</title>
<style>
#container {
width: 100%;
height: 300px;
border: 2px solid #000;
display: flex;
align-items: center;
justify-content: center;
}
button {
background-color: #09ba79;
color: white;
border-radius: 10px;
padding: 15px 32px;
cursor: pointer;
width: 250px;
height: 50px;
}
</style>
</head>
<body>
<div id="container">
<button type="button">Hit Me!</button>
</div>
</body>
</html>
Method 2. Employing Position and Transform Properties
An alternate method to center a button within a div leans on the utilization of position and transform properties. This technique centers the button vertically by setting the button’s position to relative and then using the top and transform properties.
Here’s how you can implement this method:
<div id="container">
<button class="btn" onClick="setSid()">Hit Me!</button>
</div>
#container {
position: fixed;
width: 200px;
height: 200px;
top: 50%;
left: 50%;
margin-top: -100px;
margin-left: -100px;
border: 1px solid #000;
text-align: center;
}
.btn {
position: relative;
top: 50%;
transform: translateY(-50%);
}
Method 3: Button Centering with Margin
An additional technique to center a button within a div involves manipulating the margin property of the button. This strategy involves setting both the left and right margins to auto, which allows the browser to automatically balance the margins and horizontally center the button within the div.
Here’s how it’s done:
<div style="border: 1px solid">
<button class="button" style="display: block; margin: 0 auto;">Click Me!</button>
</div>
In this example, the display property of the button is set to block which allows it to occupy the full width of its parent (div). The margin property is set to 0 auto signifies that the top and bottom margins are set to 0, while the left and right margins are automatically adjusted to center the button within the div.
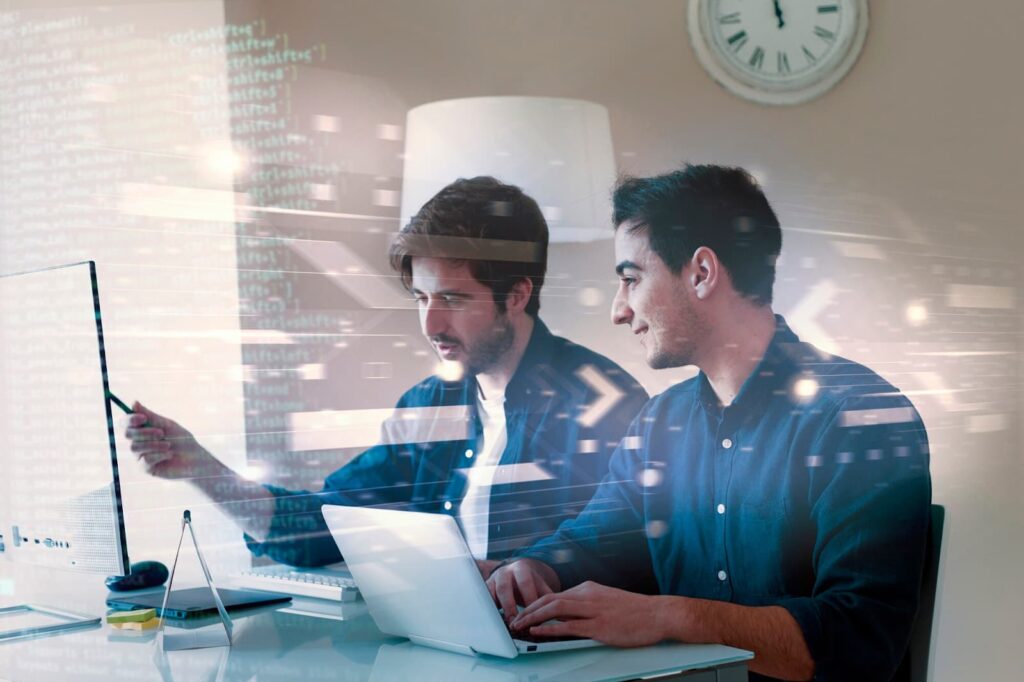
Centering a Button using CSS Flexbox
Overview of CSS Flexbox
Flexbox is a layout model in CSS that allows for the alignment and distribution of elements within a container. It provides a flexible way to achieve both vertical and horizontal centering of buttons with minimal code.
Steps to Center a Button Horizontally using Flexbox
- Create a container element to hold the button;
- Apply display: flex; to the container to activate Flexbox properties;
- Utilize justify-content: center; to horizontally center the button within the container.
Advantages of Using Flexbox for Button Centering
- Simplifies the process of aligning elements;
- Offers responsive design capabilities;
- Supports easy reordering of elements within the container.
Steps to Center Multiple Buttons using Flexbox
- Place multiple buttons within a parent container;
- Set the parent container’s display property to flex;
- Use justify-content: center; to horizontally center the buttons.
Centering a Button using CSS Grid
Exploring CSS Grid for Button Alignment
CSS Grid is a powerful layout system that enables precise control over the placement and alignment of elements on a webpage. It offers a grid-based approach to organizing content, making it ideal for centering buttons.
Steps to Center a Button Horizontally using CSS Grid
- Define a grid container to hold the button;
- Specify the number of columns and rows in the grid;
- Use place-items: center; to horizontally and vertically center the button within the grid.
Pros and Cons of CSS Grid for Button Centering
Pros | Cons |
---|---|
Allows for complex layouts | Requires a deeper understanding of grid properties and syntax. |
Provides control over element placement | May not be necessary for simpler button centering requirements. |
Supports responsive design | Compatibility issues with older browsers may arise due to advanced features. |
Centering a Button using CSS Float
Leveraging CSS Float Property for Button Alignment
While CSS Float is traditionally used for text wrapping around images, it can also be employed to center elements like buttons within a container. Although not as commonly used for centering buttons, it offers an alternative method worth exploring.
Steps to Center a Button Vertically using CSS Float
- Apply float: left; and float: right; to the button to position it within the container;
- Adjust margins and padding to fine-tune the vertical alignment of the button.
Considerations when Using CSS Float for Button Centering
- Limited support for responsive design;
- Requires additional clearing elements to prevent layout issues;
- Not recommended for complex layout requirements.
Compare and Contrast Between Various Methods of Centering a Button
When choosing a method to center buttons in CSS, it’s essential to consider factors such as ease of implementation, browser compatibility, responsiveness, and design flexibility. Each technique—Flexbox, CSS Grid, and CSS Float—offers unique advantages and limitations based on specific project requirements.
Flexbox vs. CSS Grid
- Flexbox: Ideal for simple layouts and quick alignment tasks;
- CSS Grid: Suited for complex grid-based designs and precise control over element placement.
Flexbox vs. CSS Float
- Flexbox: Provides better support for responsive design and easier alignment of multiple elements;
- CSS Float: Limited in its application, more suitable for basic alignments within a container.
CSS Grid vs. CSS Float
- CSS Grid: Offers advanced layout capabilities and responsiveness but requires a deeper understanding of grid properties;
- CSS Float: Simple to implement but lacks robust support for modern design requirements.
Endnote
Mastering the centering buttons using CSS is a valuable skill for web designers and developers. By understanding the nuances of different techniques such as Flexbox, CSS Grid, and CSS Float, you can elevate the visual appeal and user experience of your websites. Experimenting with these methods and adapting them to suit your design needs will empower you to create engaging and aesthetically pleasing button layouts.
Further Readings
For more in-depth insights into CSS layout techniques and best practices, consider exploring the following resources:
- CSS Tricks: A comprehensive guide to CSS Flexbox and Grid;
- MDN Web Docs: Detailed documentation on CSS layout models and properties;
- Smashing Magazine: Articles on modern CSS design trends and techniques.
Conclusion
In conclusion, mastering the centering buttons using CSS opens up a world of design possibilities for web developers. Whether you opt for the simplicity of Flexbox, the precision of CSS Grid, or the versatility of CSS Float, each method offers a unique approach to achieving button alignment. By leveraging these techniques effectively, you can create visually striking and user-friendly button layouts that enhance the overall aesthetics and usability of your websites. Embrace experimentation and continuous learning to stay at the forefront of modern web design practices.