With the shift towards digital accessibility on varied screen sizes, ranging from expansive desktop displays to compact mobile screens, the need for responsively resizing images using CSS has emerged as a necessary skill in web development. Cascading Style Sheets (CSS) is the coding language responsible for the aesthetic presentation of a web document.
Resizing images without compromising their quality and clarity is crucial for maintaining the integrity and authenticity of the visuals. It preserves the sharpness, details, and overall appeal of an image. Therefore, learning the resizing with CSS image size becomes an integral factor especially when posting images online. It holds paramount importance in safeguarding the professional quality and visual consistency of online content.
In this comprehensive guide, an assortment of tools to build adaptable websites with CSS image sizes is at your disposal. It aims to provide you with methods that work seamlessly across multiple screen sizes.
The Significance of Image Resizing in Web Design
Adjusting the dimensions of images is a fundamental aspect of web development, instrumental in enhancing user experience and boosting the efficiency of a website. It forms the crux of responsive web design – a design approach that aims to fabricate websites offering an excellent viewing experience across a spectrum of devices.
Why is Image Resizing Essential?
- Boosting User Experience: Bulky and non-optimized images can result in a sluggish website, drastically degrading the user experience. By tactfully resizing images, developers can assure speedy website loading, offering users a smooth and enjoyable browsing journey;
- Economizing Bandwidth and Storage: Image resizing significantly reduces the file size, thereby economizing both bandwidth and storage space – a crucial consideration for websites housing numerous high-resolution images;
- Responsive Design: Given the diversity of devices used for web access today, it is essential that images scale efficiently, irrespective of screen size. Resized images contribute to a responsive design, ensuring a visually appealing website across different devices.
Resizing an image in CSS can be achieved using the width and height properties. Consider the following simple illustration:
.image {
width: 50%;
height: auto;
}
.container {
margin: 0.5rem;
width: 91.666667%;
border-width: 2px;
border-color: #EF4444;
}
<div class="container">
<img src="flower.jpg" class="image">
</div>
<div class="container">
<img src="flower.jpg">
</div>
In this particular case, the image width is established as 50% of its containing element, while the height is kept auto to maintain the image’s aspect ratio.
For complex cases, such as wanting to cap the maximum width or height of an image, developers can utilize the max-width and max-height properties:
.image {
max-width: 100%;
height: auto;
}
.container {
margin: 0.5rem;
width: 91.666667%;
border-width: 2px;
border-color: #EF4444;
}
<div class="container">
<img src="flower.jpg" class="image">
</div>
<div class="container">
<img src="flower.jpg">
</div>
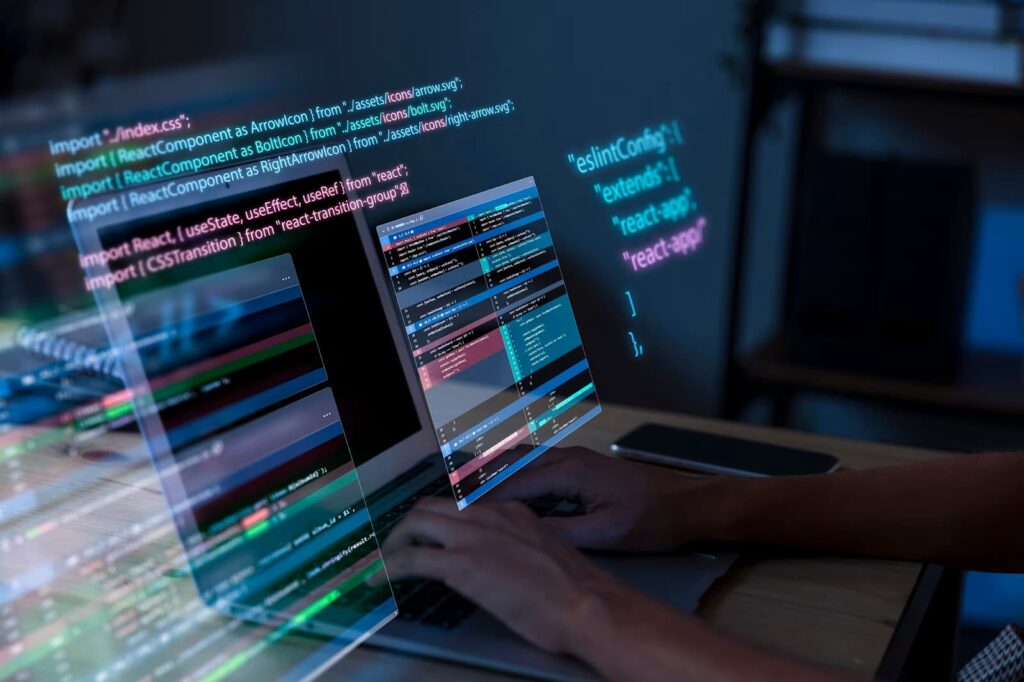
The Role of Responsive Web Development in Enhancing User Experience
Responsive web development signifies the creation of websites that dynamically adapt their layout to different screen sizes, orientations, and device types. It is crucial for providing a seamless and consistent web browsing experience. With the help of responsive techniques, a website’s appearance can be optimized for viewing through a variety of mediums, whether it be a smartphone, tablet, or desktop computer.
The Critical Need for Responsive Web Development
- Adaptability Across Devices: The modern age is characterized by a vast range of devices that vary in screen sizes and resolutions. Consequently, a website should be able to maintain its functionality and aesthetics across all these devices. Responsive design is the key to achieving a consistent user experience regardless of the device in use;
- Enhanced User Experience: A website adapts to the needs of its users through responsive design. It adjusts its layout based on the device’s size and capabilities. For instance, on a smartphone, content would be displayed in a single-column view, but on a tablet, this may change to a two-column layout;
- Cost Efficiency: Managing separate websites for desktop and mobile audiences can be financially draining. Responsive design eliminates the need for a separate mobile website, making it a financially savvy choice. A single responsive design investment ensures a website’s adaptability on all devices;
- SEO Benefits: Responsive web design not only improves user experience and reduces costs, but it also brings significant SEO advantages. High-quality backlinks and low bounce rates contribute to an improved search ranking. Mobile-optimized sites also get an added SEO benefit.
Responsive design hinges on a flexible grid system. In CSS, widths are assigned in percentages rather than pixels, which allows content to fit adaptively within its container.
.container {
width: 100%;
height: 200px;
margin: 0.5rem;
border-width: 2px;
border-color: #EF4444;
}
<div class="container"></div>
Media queries form another fundamental component of responsive design. They enable the application of distinct CSS rules for different viewport sizes.
@media screen and (max-width: 600px) {
.container {
background-color: lightblue;
margin: 0.5rem;
border-width: 2px;
border-color: #EF4444;
height: 200px;
}
}
<div class="container"></div>
Effective Techniques for Image Resizing in CSS Using the Width Property
The resizing images in CSS is a pivotal skill for creating an adaptable and optimized website. The width property is a critical component in this process as it provides the opportunity to specify the width of an image in a flexible manner, consistently across different viewing platforms.
What is Width Property?
The width property in CSS dictates the breadth of an image and can be specified using a variety of units of measurement:
- Pixels (px): This sets the width in terms of absolute pixels;
- Percentages (%): This sets the width relative to the parent container;
- Viewport Width (vw): This sets the width as a certain percentage of the total viewport width.
Choosing the appropriate unit hinges on the layout requirements of your website. Utilizing percentages or viewport units will allow the image to resize responsively, whereas pixel value results in a fixed width.
Implementing the Width Property
To resize an image using the width property, you simply need to integrate it into the CSS rule for your image. For instance:
.image {
width: 50%;
}
.container {
margin: 0.5rem;
width: 91.666667%;
border-width: 2px;
border-color: #EF4444;
}
<div class="container">
<img src="flower.jpg" class="image">
</div>
<div class="container">
<img src="flower.jpg">
</div>
Aspect Ratio Preservation
Preserving the aspect ratio while resizing images is critical to avoid distortions. You can achieve this by setting the height property to ‘auto’:
.image {
width: 50%;
height: auto;
}
In this example, the height of the image is auto-adjusted to maintain the aspect ratio based on the specified width.
Creating Responsive Images
For a fully responsive image, use the ‘max-width’ property with a value of 100%. This ensures that irrespective of the screen size, the image never exceeds its container:
.image {
max-width: 100%;
height: auto;
}
.container {
margin: 0.5rem;
width: 91.666667%;
border-width: 2px;
border-color: #EF4444;
}
<div class="container">
<img src="flower.jpg" class="image">
</div>
<div class="container">
<img src="flower.jpg">
</div>
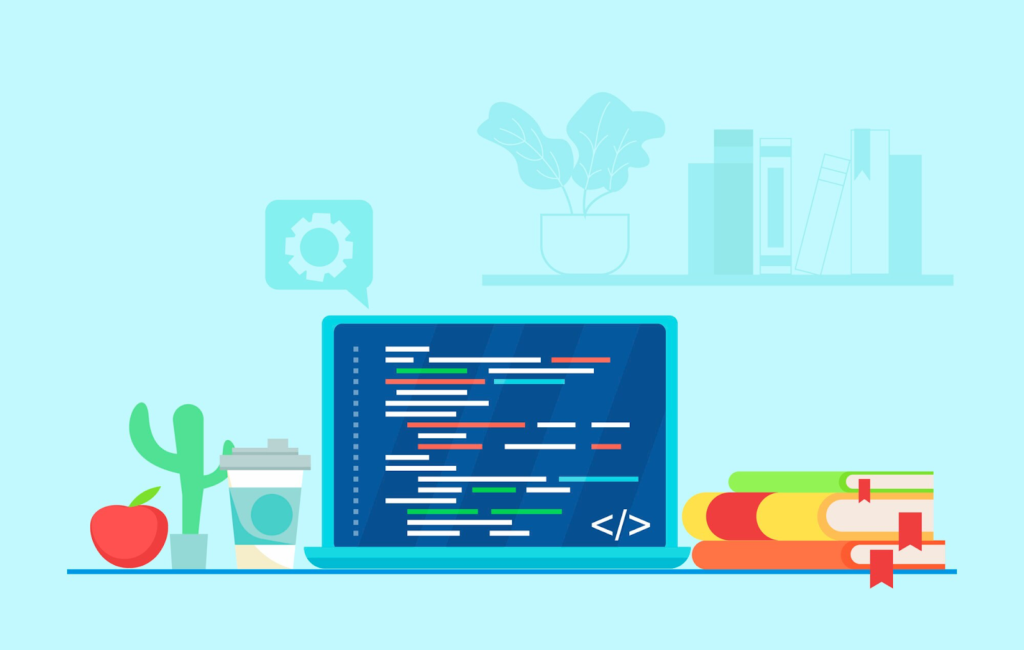
Resizing Images for Responsiveness Using CSS
Resizing images in CSS involves leveraging properties like max-width, max-height, object-fit, and background-size to ensure images adapt seamlessly to various screen sizes. Let’s explore three methods for resizing images responsively and maintaining their quality.
Method 1: Using max-width and max-height Property
The max-width and max-height properties in CSS allow you to set limits on how large an image can be displayed without distorting its aspect ratio. By defining these constraints, you ensure that images scale down proportionally to fit within specified dimensions.
Steps to Implement max-width and max-height
- Assign a max-width value to the image container to restrict the maximum width the image can occupy;
- Set a max-height value to control the maximum height of the image within the container;
- Use percentage values for max-width and max-height to enable flexible resizing based on the container’s size.
Example:
.image-container {
max-width: 100%;
max-height: 100%;
}
.image {
width: 100%;
height: auto;
}
Method 2: Using the object-fit Property
The object-fit property in CSS allows you to define how an image should be resized and positioned within its container. This property offers options such as cover, contain, fill, scale-down, and none, each catering to specific resizing requirements.
Applying object-fit for Responsive Image Display
- Choose the appropriate object-fit value based on your resizing needs;
- Apply the selected object-fit value to the image element to control its display behavior within the container;
- Test the image’s responsiveness across different screen sizes to ensure optimal rendering.
Example:
.image {
width: 100%;
height: 100%;
object-fit: cover;
}
Method 3: Using the background-size Property
When working with background images in CSS, the background-size property plays a crucial role in resizing and positioning the image within its container. By specifying values like cover, contain, or specific dimensions, you can tailor the background image’s display to suit your design requirements.
Values of background-size Property
- cover: Scales the background image to cover the entire container, cropping any excess;
- contain: Ensures the entire background image fits within the container without distortion;
- Custom Dimensions: Specify exact width and height values to resize the background image accordingly.
Example:
.container {
background-image: url('image.jpg');
background-size: cover;
background-position: center;
}
Conclusion
In conclusion, mastering the resizing images in CSS is essential for crafting visually captivating and responsive websites. By understanding the significance of setting width and height attributes, exploring different resizing methods using CSS properties, and rigorously testing image responsiveness, you can elevate the visual appeal and user experience of your web projects. Embrace these techniques and best practices to ensure that your images shine uniformly across all screen sizes, captivating audiences with their clarity and precision.